How to Fill ComboBox with Data inside the DataGridView in C# and MS Access Database
Submitted by janobe on Monday, April 29, 2019 - 21:23.
This time, I will teach you how to fill combobox with data in the datagridview in C# and MS Access Database. This is very useful most especially when you are dealing with combo box and datagridview control. In this way, you can manipulate the data inside a combobox easily.
Download the complete sourcecode and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in C#.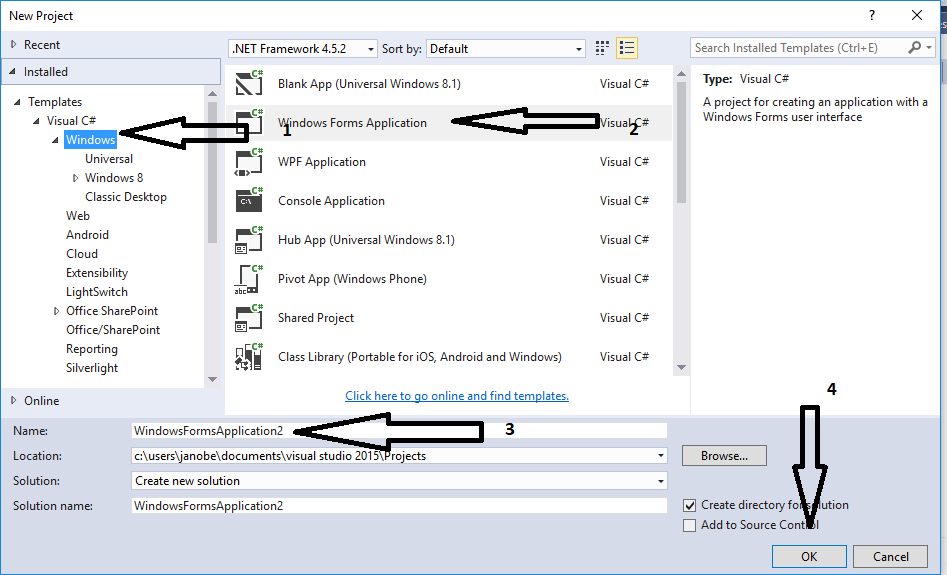
Step 2
Do the form just like shown below.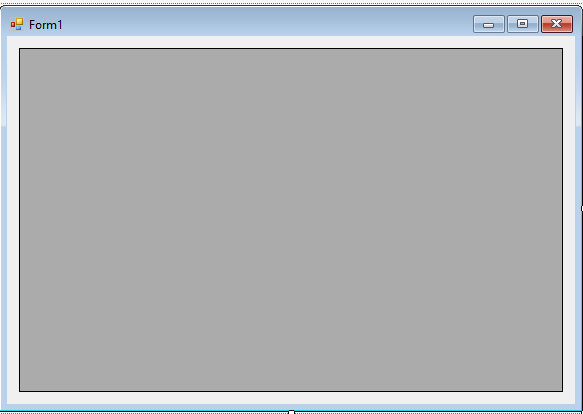
Step 3
Press F7 to open the code editor. In the code editor, add a namespace forOLeDB
to access OLeDB
libraries.
- using System.Data.OleDb;
Step 4
Create a connection between the access database and c#. After that, declare all the classes that are needed.- OleDbConnection con = new OleDbConnection("Provider = Microsoft.ACE.OLEDB.12.0; Data Source =" + Application.StartupPath + "/studentdb.accdb");
- OleDbCommand cmd;
- OleDbDataAdapter da;
- DataTable dt;
- string sql;
Step 5
Create a method for adding the comboxbox column with data in the datagridview.- private void fill_combo(string sql)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- cbo.HeaderText="Name";
- cbo.DataSource = dt;
- cbo.ValueMember = "ID";
- cbo.DisplayMember = "fname";
- dataGridView1.Columns.Add(cbo);
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Do the following codes to execute the method that you have created in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "Select * From tblstudent";
- fill_combo(sql);
- }