How to Create a Login form in Visual Basic.Net and MySQL Database
Submitted by joken on Friday, October 18, 2013 - 10:06.
In this tutorial, I'm going to show you how to create a simple Login-Logout system using Visual basic.net and MySQl Database. To start with this application, open Visual Basic->Create a New Project->Save it as “Login”.
This time, let’s add objects to our windows form and these objects are the following: four Labels, two Textbox,two buttons and a Groupbox.
This time, let’s we are now ready to add functionality to our application. To do this, double click the form and add the following code:
This code will simply disable the group box that holding the label Username and Password same with the two textbox and two Buttons.
Next, double click the “lbllogin” label and add the following code:
This code will check if the “lbllogin” is set to “Logout” then it reset the text of “lbllogin” to “Login” same with the “lblname” to “Hi, Guest!”, else if the text of “lbllogin” is equal to “Login” then it's enabled the Group box.
Then add the following code under the public class.
And double click the “OK” button and add the following code:
This time you can now test your application by pressing “F5”.
And here's the database table used.
After testing the program, here’s all the following code used in this application.
Designing object Properties
Object Property Settings Label1 Name lbllogin Text Login Label2 Name lblname Text Hi,Guest! Label3 Text Username Label4 Text Password Textbox1 Name txtuname Textbox2 Name txtpass PasswordChar * Button1 Name btnok Text OK Button2 Name btncancel Text CancelAfter setting the Object properties, arrange all the objects like as shown below.
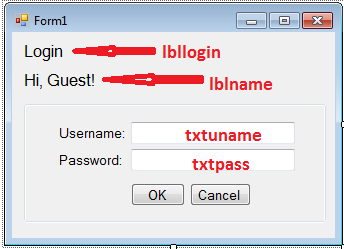
- GroupBox1.Enabled = False
- If lbllogin.Text = "Logout" Then
- lbllogin.Text = "Login"
- lblname.Text = "Hi, Guest!"
- ElseIf lbllogin.Text = "Login" Then
- GroupBox1.Enabled = True
- End If
- 'Represents an SQL statement or stored procedure to execute against a data source.
- Dim cmd As New MySqlCommand
- Dim da As New MySqlDataAdapter
- 'declare conn as connection and it will now a new connection because
- 'it is equal to Getconnection Function
- Dim con As MySqlConnection = jokenconn()
- Public Function jokenconn() As MySqlConnection
- Return New MySqlConnection("server=localhost;user id=root;password=;database=studentdb")
- End Function
- Dim sql As String
- Dim publictable As New DataTable
- Try
- 'check if the textbox is equal to nothing then it will display the message below!.
- If txtuname.Text = "" And txtpass.Text = "" Then
- Else
- sql = "select * from tbluseraccounts where username ='" & txtuname.Text & "' and userpassword = '" & txtpass.Text & "'"
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(publictable)
- 'check if theres a result by getting the count number of rows
- If publictable.Rows.Count > 0 Then
- 'it gets the data from specific column and assign to the variable
- Dim user_type, name As String
- user_type = publictable.Rows(0).Item(4)
- name = publictable.Rows(0).Item(2)
- 'check if the type of user is admin
- If user_type = "Admin" Then
- 'welcomes the user as Admiistrator
- 'set the lbllogin text to Logout
- lbllogin.Text = "Logout"
- 'disabled the groupbox
- GroupBox1.Enabled = False
- 'reset all the two textbox
- txtuname.Text = ""
- txtpass.Text = ""
- 'set the lblname to the specific user
- lblname.Text = "Hi, " & name
- ElseIf user_type = "Encoder" Then
- lbllogin.Text = "Logout"
- GroupBox1.Enabled = False
- txtuname.Text = ""
- txtpass.Text = ""
- lblname.Text = "Hi, " & name
- Else
- lbllogin.Text = "Logout"
- GroupBox1.Enabled = False
- txtuname.Text = ""
- txtpass.Text = ""
- lblname.Text = "Hi, " & name
- End If
- Else
- txtuname.Text = ""
- txtpass.Text = ""
- End If
- da.Dispose()
- End If
- Catch ex As Exception
- End Try
- con.Clone()
- --
- -- Dumping data for table `tbluseraccounts`
- --
- (1, 'admin', 'Joken Villanueva', 'admin123', 'Admin'),
- (2, 'jason', 'Jason Batuto', 'jason123', 'Encoder');
- Imports MySql.Data.MySqlClient
- Public Class Form1
- 'Represents an SQL statement or stored procedure to execute against a data source.
- Dim cmd As New MySqlCommand
- Dim da As New MySqlDataAdapter
- 'declare conn as connection and it will now a new connection because
- 'it is equal to Getconnection Function
- Dim con As MySqlConnection = jokenconn()
- Public Function jokenconn() As MySqlConnection
- Return New MySqlConnection("server=localhost;user id=root;password=;database=studentdb")
- End Function
- Private Sub btnlogin_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnlogin.Click
- Dim sql As String
- Dim publictable As New DataTable
- Try
- 'check if the textbox is equal to nothing then it will display the message below!.
- If txtuname.Text = "" And txtpass.Text = "" Then
- Else
- sql = "select * from tbluseraccounts where username ='" & txtuname.Text & "' and userpassword = '" & txtpass.Text & "'"
- 'bind the connection and query
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(publictable)
- 'check if theres a result by getting the count number of rows
- If publictable.Rows.Count > 0 Then
- 'it gets the data from specific column and assign to the variable
- Dim user_type, name As String
- user_type = publictable.Rows(0).Item(4)
- name = publictable.Rows(0).Item(2)
- 'check if the type of user is admin
- If user_type = "Admin" Then
- 'welcomes the user as Admiistrator
- 'set the lbllogin text to Logout
- lbllogin.Text = "Logout"
- 'disabled the groupbox
- GroupBox1.Enabled = False
- 'reset all the two textbox
- txtuname.Text = ""
- txtpass.Text = ""
- 'set the lblname to the specific user
- lblname.Text = "Hi, " & name
- ElseIf user_type = "Encoder" Then
- lbllogin.Text = "Logout"
- GroupBox1.Enabled = False
- txtuname.Text = ""
- txtpass.Text = ""
- lblname.Text = "Hi, " & name
- Else
- lbllogin.Text = "Logout"
- GroupBox1.Enabled = False
- txtuname.Text = ""
- txtpass.Text = ""
- lblname.Text = "Hi, " & name
- End If
- Else
- txtuname.Text = ""
- txtpass.Text = ""
- End If
- da.Dispose()
- End If
- Catch ex As Exception
- End Try
- con.Clone()
- End Sub
- Private Sub lbllogin_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles lbllogin.Click
- If lbllogin.Text = "Logout" Then
- lbllogin.Text = "Login"
- lblname.Text = "Hi, Guest!"
- ElseIf lbllogin.Text = "Login" Then
- GroupBox1.Enabled = True
- End If
- End Sub
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- GroupBox1.Enabled = False
- End Sub
- End Class
Comments
Small Problem
I'm thinking line 5
If txtuname.Text = "" And txtpass.Text = "" Then
should Be
If txtuname.Text = "" Or txtpass.Text = "" Then
That way it would match your comment and properly test for null strings.
Small Problem
I'm thinking line 5
If txtuname.Text = "" And txtpass.Text = "" Then
should Be
If txtuname.Text = "" Or txtpass.Text = "" Then
That way it would match your comment and properly test for null strings.