Learning More About Functions
Submitted by Muzammil Muneer on Sunday, September 21, 2014 - 16:08.
Learning More about Functions
In this part you will learn: 1. C syntax 2. Functions 3. Function Calling 4. Function definition 5. Function prototype 6. Recursive Functions 7. Showing outputWhat is Recursion?
Recursion is a process of repeating of items in a self-similar way. A recursive function is a function that calls itself and this technique is known as recursion in C programming. Recursion helps to make functions precise and easy. Recursive Function Basic Step: Open Dev C++ then File > new > source file and start writing the code below.- #include<stdio.h>
- #include<conio.h>
In this program we are going to calculate the factorial of number that has been entered by the user using recursion. We will write a recursive function that will keep on calling itself until a certain condition is met.
First of all we declared the function prototype. The function takes one parameter which is an integer and returns a integer.
Then we started our main function. In this function we declared a integer variable and took input from the user in that variable. After that we passed that variable to the function.
Note that when we pass a variable to a function it is passed by value. This means another copy of that variable is made in the memory with same value but different name. Similarly when a variable is declared in any function the scope of the variable is that function. This means that when the program exits that particular function that variable is deleted from the memory.
The factorial function returns us the answer of the calculation and then we store that answer in a new variable. At the end we print the answer and pauses our program
- int factorial(int a)
- {
- if(a==0)
- {
- return 1;
- }
- else
- return a*factorial(a-1);
- }
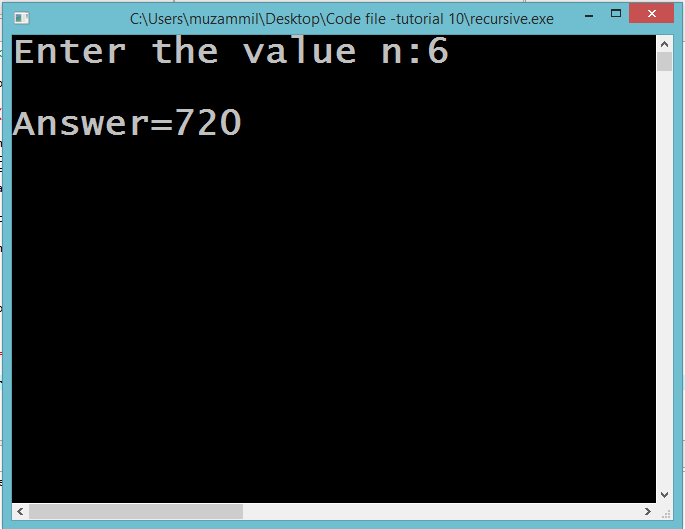