Data structures are fundamental components in computer science that allow us to store, organize, and manage data efficiently. They provide a way to structure and store information, making it accessible and manageable for various computational tasks. Data structures come in various types, each with its own set of rules and methods for accessing and manipulating the data they hold. Whether it's arrays, linked lists, trees, or more complex structures like graphs and hash tables, choosing the right data structure for a specific problem is crucial for optimizing performance and solving complex computational challenges. Understanding data structures is essential for anyone working in software development or computer science, as they form the basis for building and designing efficient algorithms and applications.
Data structures are the building blocks that help us organize and manage data effectively. Whether you're a seasoned programmer or just starting your journey into the world of coding, understanding these fundamental data structures is essential. In this comprehensive guide, we'll take you on a journey to explore the basics of data structures. We'll break down these concepts in a simple and accessible way, so you can grasp the core ideas without getting lost in jargon or complexity. So, let's dive in and discover the essential data structures that are the backbone of software development.
There are various types of data structures, each with its own characteristics and use cases. Here are some of the most common types of data structures:
1. Arrays
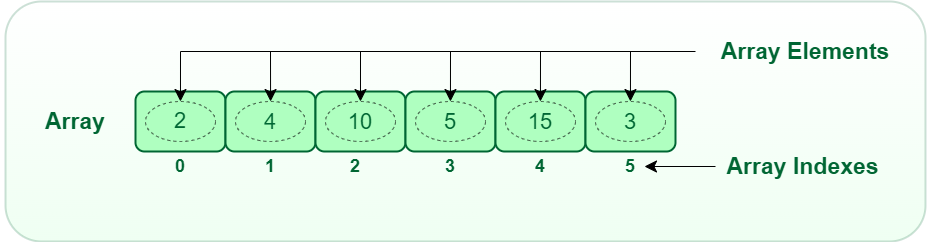
- An array is like a list where you can keep multiple things in order. Imagine it as a row of boxes, and you can put something (like numbers or names) in each box. You can easily find what's in a specific box by specifying the box number you want to look at. It's like having a bunch of slots to store things, and you can quickly grab what you need by knowing its position in the row.
- These data structures are usually used for:
- Storing a list of elements, like numbers or names.
- Quickly accessing data using an index.
2. Linked Lists
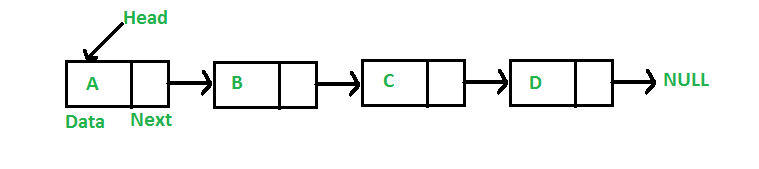
- A linked list is like a chain of containers, where each container holds something (like a number or a word) and points to the next container in the chain. It's a way to keep things in order, one after the other. This makes it easy to add or remove items from the list without moving everything around. In simple terms, it's like a string of train cars, with each car carrying a package and connected to the next car. You can add or remove cars from the train without affecting the rest of the cars.
- This type of data structure is usually used for:
- Building simple databases.
- Efficiently inserting and deleting items.
3. Stacks
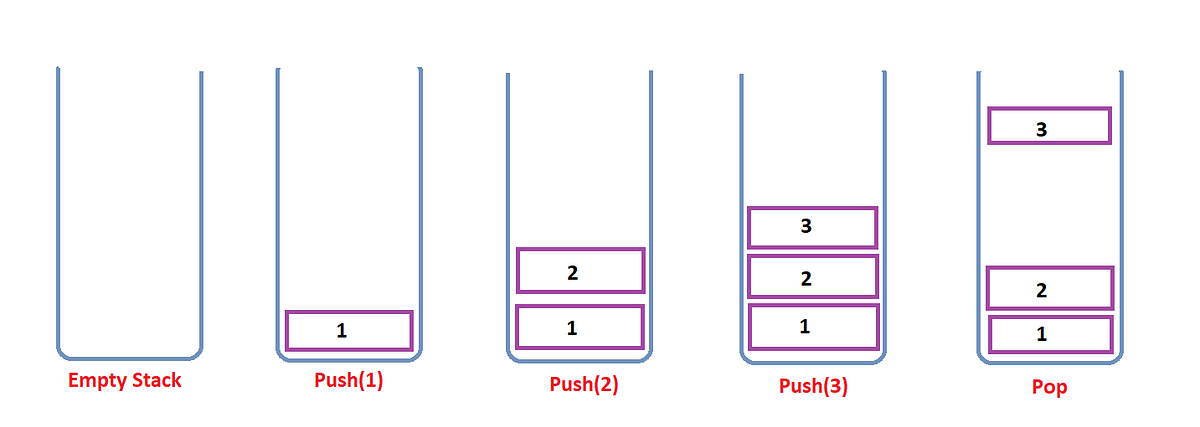
- Think of a stack like a stack of plates. You can only add or take away plates from the top. It works the same way in a computer. You put things on the "stack" (like tasks to do) one at a time, and you can only deal with the one on top. When you're done with that task, you take it off the top and deal with the next one below. This is called "Last-In-First-Out" or LIFO. Stacks are handy for keeping track of things in order, like tracking which functions your computer program needs to go back to after it finishes what it's doing now.
- This type of data structure is usually used for:
- Managing a history of actions in software.
- Solving problems in a "last in, first out" order.
4. Queues
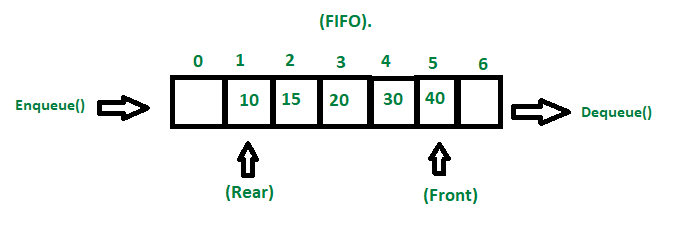
- A queue is like a line of people waiting for something. The first person who arrives is the first to get served. It's a data structure that follows this rule: "First-In-First-Out" (FIFO). Whatever goes in first, comes out first. People at the front of the line get helped before those at the back. Queues are used in computer programs for tasks that need to be done in order, like printing documents or processing orders in an online store.
- This type of data structure is usually used for:
- Handling tasks or requests in order.
- Simulating waiting lines, like at a store.
5. Trees
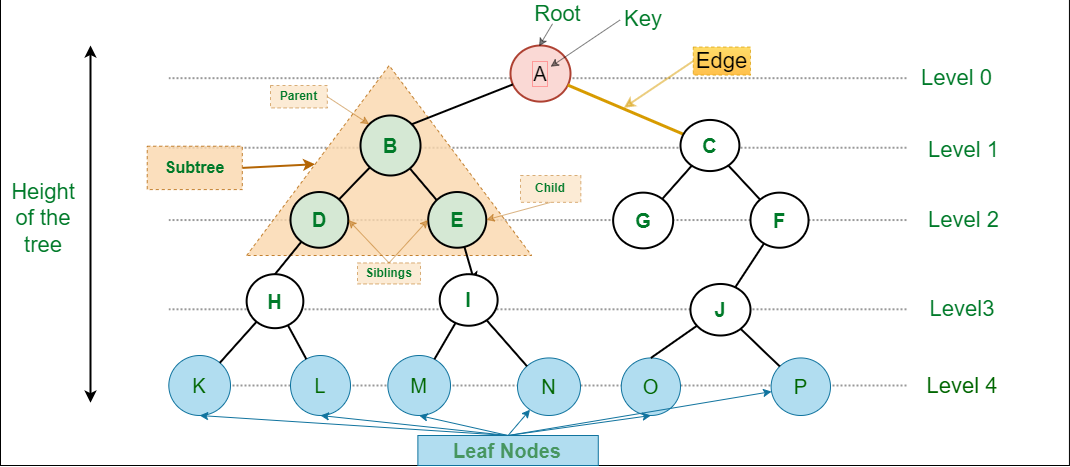
- A tree data structure is like a family tree. At the top, there's one person (the "root"). From that person, there are branches leading to others (their "children"). Those children can have their own children, and so on. Each person in the family tree is like a "node" in a tree data structure, and the connections between them are like "edges." Trees are used in computer science to organize and manage data in a hierarchical way. They help with tasks like storing files on a computer, organizing information, and searching for things quickly.
- This type of data structure is usually used for:
- Organizing hierarchical data, like folders on a computer.
- Efficiently searching and sorting information.
6. Graphs
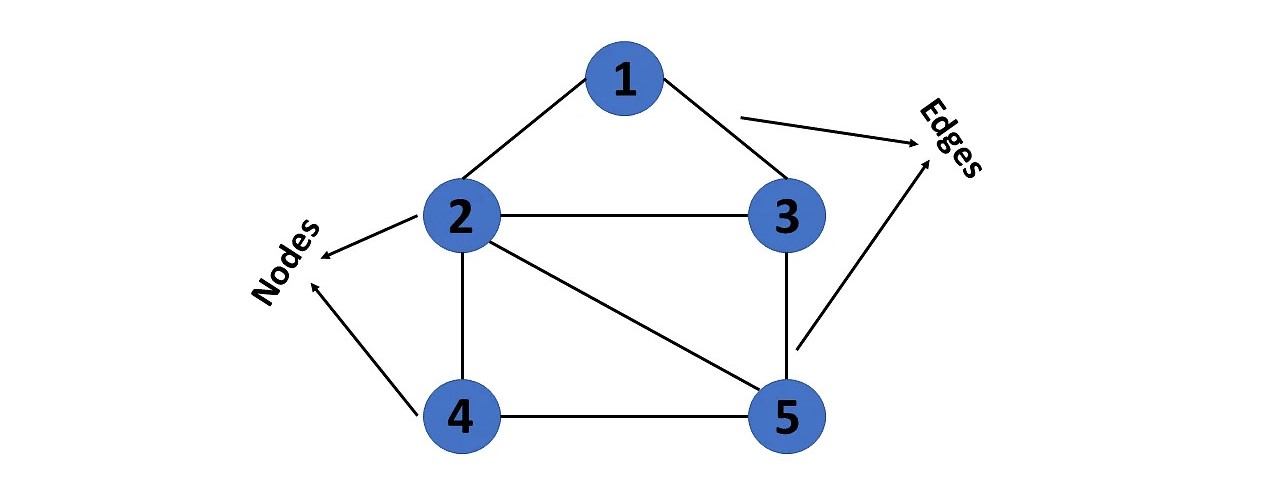
- A graph data structure is like a visual map of connections between different things. Imagine these things as points (nodes), and the connections between them as lines (edges). You can use a graph to show relationships between people, cities, websites, or any other objects. In simple words, a graph helps us see how different things are connected to each other. It's like drawing a map of friendships on a piece of paper, with each person as a point and lines showing who is friends with whom. This can be very useful for solving problems like finding the quickest route between two places or understanding how things are related in a network.
- This type of data structure is usually used for:
- Modeling connections between things, like social networks.
- Solving routing and optimization problems.
7. Hash Tables
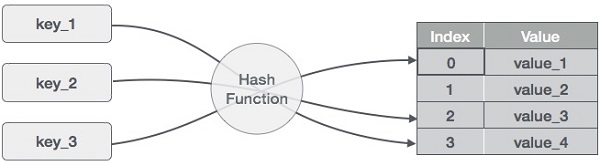
- A hash data structure is like a magical box that helps you quickly find stuff. It works like a treasure map where each item has a unique clue that leads you straight to it. So, when you need something, you use its clue (called a "key") to open the box and grab it super-fast. It's a clever way to store and find things without searching all over the place.
- This type of data structure is usually used for:
- Building dictionaries and lookup tables.
- Speedy access to data using keys.
8. Heaps
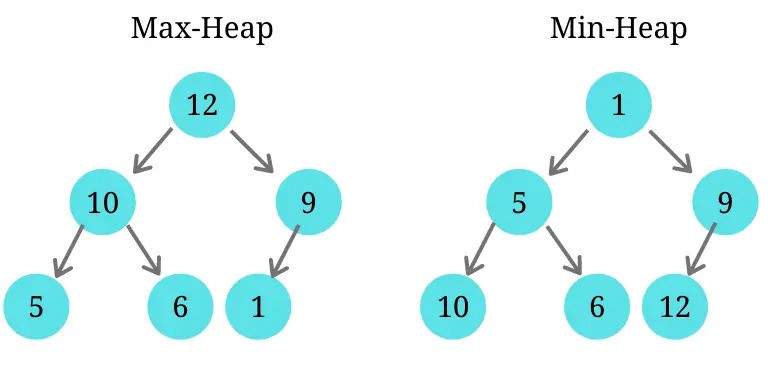
- A heap is like a special kind of list where the most important item is always at the top. Imagine a to-do list where you have to do the most urgent task first. The top item on the list is the most important thing you need to do right now. Heaps are great for managing tasks or jobs in order of importance. You can quickly figure out which task is the most crucial, and you can also add or remove tasks efficiently. So, they help you stay organized and tackle the most critical stuff first.
- This type of data structure is usually used for:
- Managing priority in tasks or jobs.
- Efficiently finding and removing the highest or lowest priority item.
9. Tries (Prefix Trees)
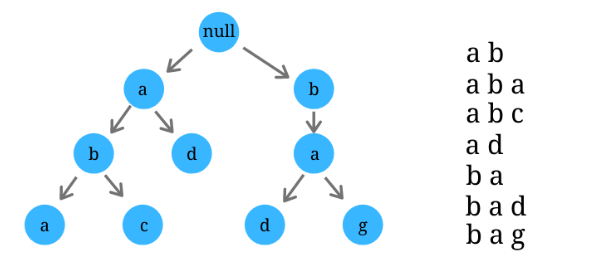
- A Trie, which sounds like "try," is a way to store and search for words or sequences of characters efficiently. Imagine it as a tree-like structure where each branch represents a letter in a word. This helps you find words quickly, like when you type a search query, and the computer suggests words starting with the letters you've typed. It's good for things like dictionaries, autocomplete, and efficient searching of words or phrases.
- This type of data structure is usually used for:
- Implementing autocomplete in search bars.
- Efficiently searching and storing words and phrases.
10. Sets and Maps
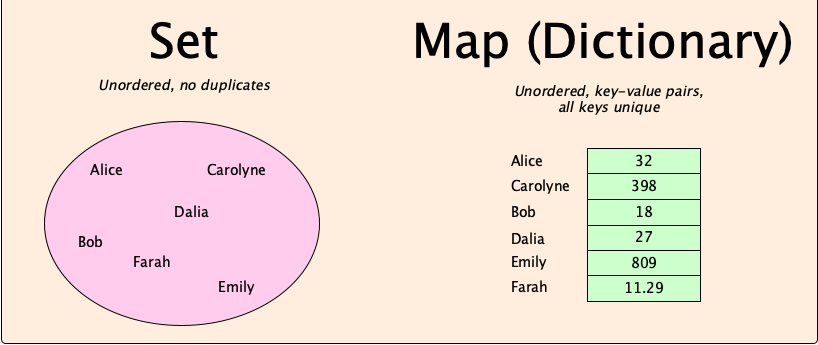
- Sets and maps are data structures that help us organize and access information. Sets are like a collection of unique items, ensuring that no two items are the same. It's handy when you need to keep track of a list of distinct things, like a list of friends' names without any duplicates. Maps, on the other hand, work like dictionaries, where you can connect one thing to another. For instance, you can associate a person's name with their phone number, making it easy to find someone's contact details. So, sets help us manage unique items, while maps help us link things together for quick and easy retrieval.
- This type of data structure is usually used for:
- Keeping track of unique items, like a list of emails.
- Storing key-value pairs for easy retrieval.
In conclusion, the world of computer science and software development is intricately woven with the fundamental types of data structures. These structures provide the building blocks that empower programmers and developers to efficiently organize, store, and manipulate data, enabling the creation of powerful and optimized applications. Our comprehensive guide has taken you on a journey through the core data structures, breaking down complex concepts into simple, accessible terms, ensuring that whether you are a seasoned coder or a novice, you can grasp the essential concepts without being overwhelmed by jargon or complexity.
We've explored an array of data structures, from the straightforward yet versatile arrays to the intricate and interconnected graphs. Each structure serves a unique purpose, making them invaluable tools for solving a wide range of computational challenges. Whether you need to manage a list of elements, prioritize tasks, model complex relationships, or ensure efficient data retrieval, there is a data structure tailored to the task at hand.
Understanding these data structures is not just a matter of academic curiosity; it is a fundamental skill for anyone involved in software development or computer science. Data structures are at the heart of designing efficient algorithms and applications, and the choice of the right data structure can significantly impact the performance of your software.
As you embark on your journey in the world of coding and software development, remember that mastering data structures is akin to learning the language of computers. These structures will be your allies in crafting efficient, elegant solutions to real-world problems. So, whether you're diving into your first lines of code or have already crafted complex applications, the knowledge of data structures will be your constant companion, supporting you on your path to becoming a proficient and skilled programmer.