if and if...else(one way selection)
Submitted by GeePee on Saturday, May 16, 2015 - 00:18.
The following is a simple Java application program that uses if and if…else(one way selection). There are only two logical values, true and false, they are extremely useful because they permit programs to incorporate decision making that alters the processing flow. . I will be using the JCreator IDE in developing the program.
Note the elements of this syntax. It begins with the reserved word
Sample run:
The statement displays the message dialog box and shows the original number, stored in
- if (expression)
- statement
if
, followed by an expression contained within parentheses, followed by a statement. The expression is sometimes called the decision maker because it decides whether to execute the statement that follows it.
The expression is logical expression, if the value of the expression is true, the statement executes, if the value is false, the statement does not execute and the computer goes on to the next statement in the program.
Here is the sample program that uses if and if…else (one way selection)
To start in this tutorial, first open the JCreator IDE, click new and paste the following code.
- import javax.swing.JOptionPane;
- public class AbsoluteValue
- {
- {
- int number;
- int temp;
- String numString;
- temp=number;
- if(number < 0)
- number = -number;
- + " is " + number,
- "Absolute Value",
- }
- }
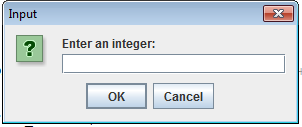
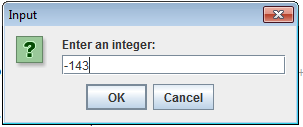
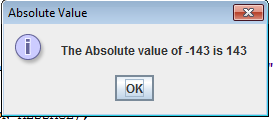
numString = JOptionPane.showInputDialog("Enter an integer:");
displays the input dialog box and prompts the user to enter an integer. The entered number is stored as a string numString.
The statement number = Integer.parseInt(numString);
uses the method parseInt
of the class Integer, converts the value of numString
into the number and stores the number in the variable number.
The statement temp=number;
copies the value of number into temp.
The statement if(number < 0)
checks whether number is negative. If the number is negative, the statementnumber = -number;
changes number to positive number.
The statement - + " is " + number,
- "Absolute Value",
temp
, and the absolute value of the number stored in number.
The statement System.exit(0);
terminates the program.