Change User Interface(UI) in Java
- import java.awt.event.*; //used to access the ActionEvent and ActionListener class
- import java.awt.*; //used to access the GridLayout class
- import javax.swing.*; //used to access the JButton,JList,JTextArea,JFrame,JPanel,SwingUtilities and UIManager class
- plaf = actionEvent.getActionCommand();
- try {
- }
- }
- };
- frame.getContentPane().add(comboBox);
- frame.getContentPane().add(txtArea);
- frame.getContentPane().add(panel);
- frame.setSize(580, 250);
- frame.setVisible(true);
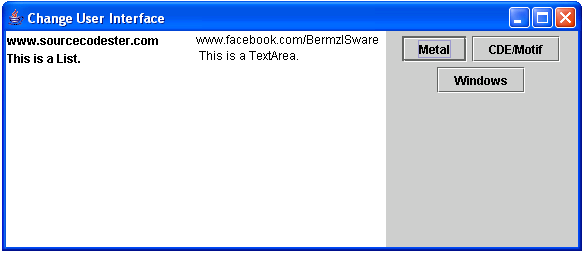
- import java.awt.event.*; //used to access the ActionEvent and ActionListener class
- import java.awt.*; //used to access the GridLayout class
- import javax.swing.*; //used to access the JButton,JList,JTextArea,JFrame,JPanel,SwingUtilities and UIManager class
- public class UILookAndFeel {
- plaf = actionEvent.getActionCommand();
- try {
- }
- }
- };
- for (int i = 0, n = UIlooks.length; i < n; i++) {
- button.setActionCommand(UIlooks[i].getClassName());
- button.addActionListener(changeUI);
- panel.add(button);
- }
- frame.getContentPane().add(comboBox);
- frame.getContentPane().add(txtArea);
- frame.getContentPane().add(panel);
- frame.setSize(580, 250);
- frame.setVisible(true);
- }
- }
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.